Academy Test Environment
Adding Context
Install Visual Studio
We build all of our applications within Visual Studio, so if you haven't got that, then download it from https://visualstudio.microsoft.com/downloads/
We wrote this in Visual Studio 2019, using the language Visual Basic, and times move so quickly that there may be parts of the articles that don't apply, or aren't in the same format as you see if using a different version.
Minimum items to install;
- ASP.NET and web development
- .NET cross-platform development
When using newer versions of Visual Studio then make sure you install the .NET Framework 4.7.2 targeting pack and the highest number SDK and Targeting Pack from the individual components tab to ensure that the templates required are installed.
Once you have downloaded and installed, then you should be able to follow the rest of the articles.
Create New Project
Name your project
Items to Add
Install SQL Server Express
The install can be done using all of the standard settings.
Keep a copy of your connection string as highlighted in the screenshot to the right, you will need it later.
You can also get a copy of SQL Server Management Studio by clicking on the "Install SSMS" button from here.
Install SQL Server Management Studio
SQL Server Management Studio can be found at https://docs.microsoft.com/en-us/sql/ssms/download-sql-server-management-studio-ssms?view=sql-server-ver15, you will be looking for the section "Free Download for SQL Server Management Studio (SSMS)" in the main body of the page.
This program can be installed with all of the default settings.
Once installed, you can then open it up, it can be found under Microsoft SQL Server Tools, and then SQL Management Studio
The database connection on the first popup should be available under "localhost\SQLEXPRESS".
Once connected, select "New Query", and use the following command to create a database "CREATE DATABASE ClaytabaseAcademy"
You can use any other databases or servers in your IT estate, you will just need to adapt code as necessary to fit any articles.
Edit the web.config file in Visual Studio
In Visual Studio, find your Web.config file and modify the contents of the <configuration> to the snippet below.
We are also adding references for MVC and a couple of other modules at this stage and setting the .NET version.
Config Settings
<configuration> <connectionStrings> <add name="SqlConnection" connectionString="Server=localhost\SQLEXPRESS;Database=ClaytabaseAcademy;Trusted_Connection=True;" providerName="System.Data.SqlClient"/> </connectionStrings> <system.web> <compilation debug="true" strict="false" explicit="true" targetFramework="4.7.2"> <assemblies> <add assembly="System.Numerics, Version=4.0.0.0, Culture=neutral, PublicKeyToken=B77A5C561934E089"/> <add assembly="System.Windows.Forms, Version=4.0.0.0, Culture=neutral, PublicKeyToken=B77A5C561934E089"/> <add assembly="System.Xml.Serialization, Version=4.0.0.0, Culture=neutral, PublicKeyToken=B77A5C561934E089"/> <add assembly="System.Net, Version=4.0.0.0, Culture=neutral, PublicKeyToken=B03F5F7F11D50A3A"/> <add assembly="System.Net.Http, Version=4.2.0.0, Culture=neutral, PublicKeyToken=B03F5F7F11D50A3A"/> <add assembly="System.Core, Version=4.0.0.0, Culture=neutral, PublicKeyToken=B77A5C561934E089"/> <add assembly="System.IdentityModel, Version=4.0.0.0, Culture=neutral, PublicKeyToken=B77A5C561934E089"/> <add assembly="System.Runtime.Serialization, Version=4.0.0.0, Culture=neutral, PublicKeyToken=B77A5C561934E089"/> <add assembly="System.Web.Routing, Version=4.0.0.0, Culture=neutral, PublicKeyToken=31BF3856AD364E35"/> <add assembly="WindowsBase, Version=4.0.0.0, Culture=neutral, PublicKeyToken=31BF3856AD364E35"/> </assemblies> </compilation> <httpRuntime targetFramework="4.7.2" /> </system.web></configuration>
Add an ASP.NET folder
Create a Public Class to share the connection string easily
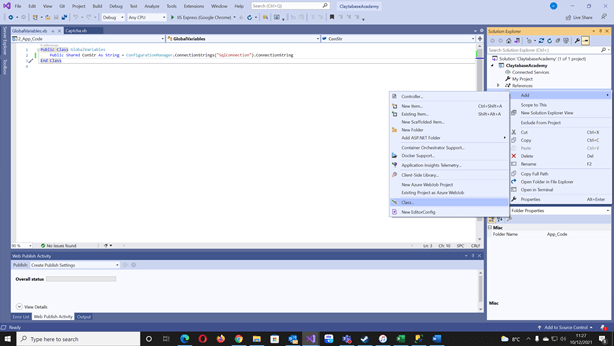
For ease of use, we can now create a Public Class to easily reference the SQL Connection in code elsewhere.
To do this, right click on the newly created App_Code folder and select Add > Class, we will call this GlobalVariables. The content of this file will be updated with the ConStr element below that we will use in other articles.
Public Class GlobalVariables Public Shared ConStr As String = ConfigurationManager.ConnectionStrings("SqlConnection").ConnectionStringEnd Class
This is also a useful place for any other shared information you may want to share.
A Gotcha: Once added, right mouse button on this item and select properties, change the Build Action to Compile
Create a Global.asax file
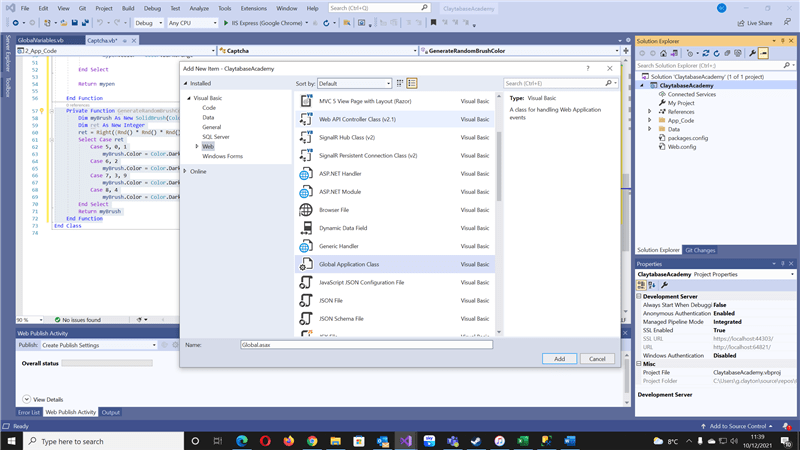
We will go through more detail in a separate article, but add a Global class to control your application.
To do this right click on Claytabase Academy and select Add > New Item and look for Global Application Class within the Web folder, leave the name name as Global.asax.
This file will need to be modified with the following;
- Import System.Web.Routing so that we can access the routing features
- Add a new sub, RegisterRoutes, in which we can add all of our dynamic routes further down the line
- Reference this new RegisterRoutes sub in the Application start, so that it gets loaded straight away.
Full code is set out below;
New Code
Imports System.Web.SessionStateImports System.Web.RoutingPublic Class Global_asax Inherits System.Web.HttpApplication Sub Application_Start(ByVal sender As Object, ByVal e As EventArgs) ' Fires when the application is started RegisterRoutes(RouteTable.Routes) End Sub Sub Session_Start(ByVal sender As Object, ByVal e As EventArgs) ' Fires when the session is started End Sub Sub Application_BeginRequest(ByVal sender As Object, ByVal e As EventArgs) ' Fires at the beginning of each request End Sub Sub Application_AuthenticateRequest(ByVal sender As Object, ByVal e As EventArgs) ' Fires upon attempting to authenticate the use End Sub Sub Application_Error(ByVal sender As Object, ByVal e As EventArgs) ' Fires when an error occurs End Sub Sub Session_End(ByVal sender As Object, ByVal e As EventArgs) ' Fires when the session ends End Sub Sub Application_End(ByVal sender As Object, ByVal e As EventArgs) ' Fires when the application ends End Sub Sub RegisterRoutes(ByVal Routes As RouteCollection)
End SubEnd Class